Note
Go to the end to download the full example code.
TDS Radar Query Service
Use Siphon to get NEXRAD Level 3 data from a TDS.
from datetime import datetime, timezone
import matplotlib.pyplot as plt
import numpy as np
from siphon.cdmr import Dataset
from siphon.radarserver import get_radarserver_datasets, RadarServer
First, point to the top-level thredds radar server accessor to find what datasets are available.
ds = get_radarserver_datasets('http://thredds.ucar.edu/thredds/')
print(list(ds))
['NEXRAD Level II Radar for Case Study CCS039', 'NEXRAD Level II Radar from IDD', 'NEXRAD Level III Radar for Case Study CCS039', 'NEXRAD Level III Radar from IDD', 'TDWR Level III Radar from IDD']
Now create an instance of RadarServer to point to the appropriate radar server access URL. This is pulled from the catalog reference url.
url = ds['NEXRAD Level III Radar from IDD'].follow().catalog_url
rs = RadarServer(url)
Look at the variables available in this dataset
print(rs.variables)
{'NBC', 'NXG', 'NVL', 'N3B', 'N3K', 'N1S', 'NAG', 'NAK', 'NYK', 'N3Q', 'N3U', 'NZQ', 'NBH', 'NZX', 'DOD', 'NTP', 'N1Q', 'NMD', 'DTA', 'NXB', 'NBB', 'DHR', 'N0R', 'N0S', 'DSP', 'N0X', 'PTA', 'N1P', 'NVW', 'NXH', 'NCR', 'N1U', 'N1H', 'NAU', 'DU6', 'NET', 'NXU', 'N3S', 'N1M', 'NZG', 'N0G', 'N2K', 'DAA', 'NXM', 'N1K', 'NAH', 'NAC', 'NZM', 'NYU', 'N0U', 'NXQ', 'NYG', 'NYC', 'EET', 'DVL', 'N1G', 'N1X', 'NYB', 'NST', 'NZB', 'N3H', 'NBM', 'DU3', 'N0V', 'NYH', 'N2Q', 'DSD', 'N0M', 'N0Q', 'N1C', 'N3M', 'N0B', 'NAX', 'N2C', 'NBQ', 'NZU', 'NBK', 'N2S', 'NZK', 'NXX', 'N2B', 'N0K', 'N0Z', 'N0C', 'NZC', 'NAQ', 'NAM', 'N2M', 'HHC', 'NYM', 'NAB', 'NYX', 'NBX', 'NXK', 'N3X', 'N2U', 'N2X', 'N2H', 'NYQ', 'N0H', 'N1B', 'DPR', 'DPA', 'OHA', 'NZH', 'N3C', 'NBU', 'NXC'}
Create a new query object to help request the data. Using the chaining methods, ask for data from radar CYS (Cheyenne) for now for the product N0B, which is reflectivity data for the lowest tilt. We see that when the query is represented as a string, it shows the encoded URL.
query = rs.query()
query.stations('CYS').time(datetime.now(timezone.utc)).variables('N0B')
var=N0B&time=2025-01-24T20%3A05%3A55.564916%2B00%3A00&stn=CYS
We can use the RadarServer instance to check our query, to make sure we have required parameters and that we have chosen valid station(s) and variable(s)
True
Make the request, which returns an instance of TDSCatalog. This handles parsing the catalog
We can look at the datasets on the catalog to see what data we found by the query. We find one NIDS file in the return.
print(catalog.datasets)
['Level3_CYS_N0B_20250124_2000.nids']
We can pull that dataset out of the dictionary and look at the available access URLs. We see URLs for OPeNDAP, CDMRemote, and HTTPServer (direct download).
ds = list(catalog.datasets.values())[0]
print(ds.access_urls)
{'OPENDAP': 'https://thredds.ucar.edu/thredds/dodsC/nexrad/level3/IDD/N0B/CYS/20250124/Level3_CYS_N0B_20250124_2000.nids', 'HTTPServer': 'https://thredds.ucar.edu/thredds/fileServer/nexrad/level3/IDD/N0B/CYS/20250124/Level3_CYS_N0B_20250124_2000.nids', 'CdmRemote': 'https://thredds.ucar.edu/thredds/cdmremote/nexrad/level3/IDD/N0B/CYS/20250124/Level3_CYS_N0B_20250124_2000.nids'}
We’ll use the CDMRemote reader in Siphon and pass it the appropriate access URL.
data = Dataset(ds.access_urls['CdmRemote'])
The CDMRemote reader provides an interface that is almost identical to the usual python NetCDF interface. We pull out the variables we need for azimuth and range, as well as the data itself.
rng = data.variables['gate'][:] / 1000.
az = data.variables['azimuth'][:]
ref = data.variables['BaseReflectivityDR'][:]
Need to adjust range and azimuth so that they bound the range gates rather than having a single point at the start of the gate.
Then convert the polar coordinates to Cartesian
x = rng * np.sin(np.deg2rad(az))[:, None]
y = rng * np.cos(np.deg2rad(az))[:, None]
ref = np.ma.array(ref, mask=np.isnan(ref))
Finally, we plot them up using matplotlib.
fig, ax = plt.subplots(1, 1, figsize=(9, 8))
ax.pcolormesh(x, y, ref)
ax.set_aspect('equal', 'datalim')
ax.set_xlim(-460, 460)
ax.set_ylim(-460, 460)
plt.show()
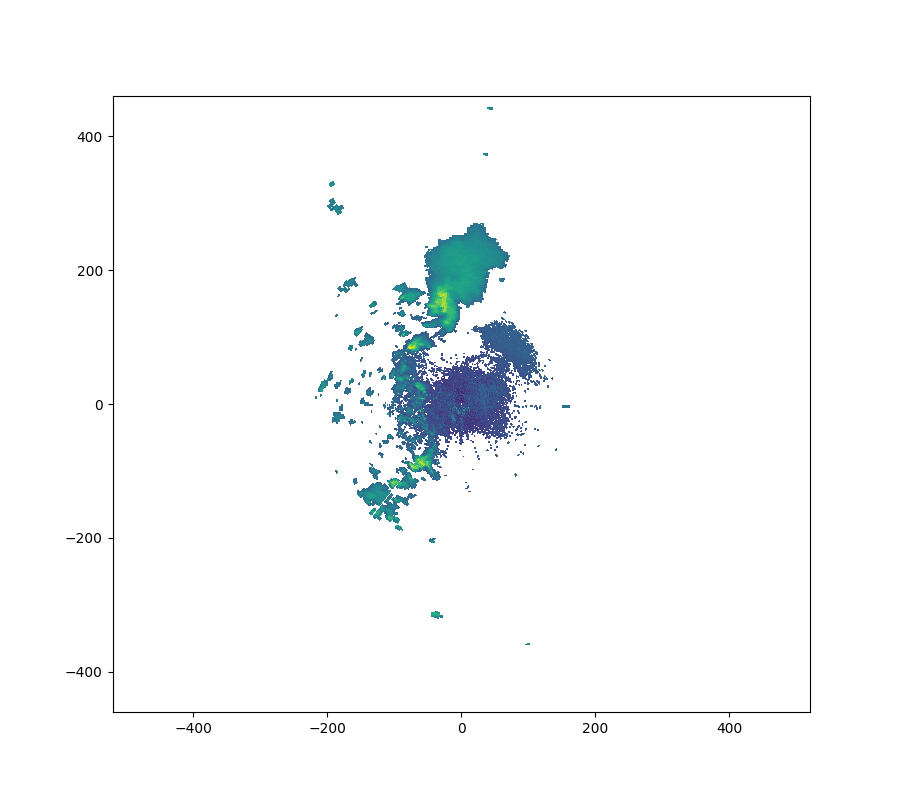
Total running time of the script: (0 minutes 2.492 seconds)