Plotting and Interactivity
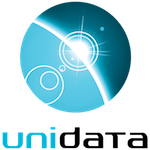
Plotting and Jupyter Notebooks
Unidata Python Workshop
One of the most common tasks we face as scientists is making plots. Visually assessing data is one of the best ways to explore it - who can look at a wall of tabular data and tell anything? In this lesson we'll show how to make some basic plots in notebooks and introduce interactive widgets.
Matplotlib has many more features than we could possibly talk about - this is just a taste of making a basic plot. Be sure to browse the matplotlib gallery for ideas, inspiration, and a sampler of what's possible.
In [1]:
# Import matplotlib as use the inline magic so plots show up in the notebook
import matplotlib.pyplot as plt
%matplotlib inline
In [2]:
# Make some "data"
x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
y = [2, 4, 8, 16, 32, 64, 128, 256, 512, 1024]
Basic Line and Scatter Plots¶
In [3]:
# Make a simple line plot
plt.plot(x, y)
Out[3]:
In [4]:
# Play with the line style
plt.plot(x, y, color='tab:red', linestyle='--')
Out[4]:
In [5]:
# Make a scatter plot
plt.plot(x, y, color='tab:orange', linestyle='None', marker='o')
Out[5]:
Adding Interactivity to Plots¶
In [6]:
# Let's make some more complicated "data" using a sine wave with some
# noise superimposed. This gives us lots of things to manipulate - the
# amplitude, frequency, noise amplitude, and DC offset.
import numpy as np
x = np.linspace(0, 2*np.pi, 100)
y = 10 * np.sin(x) + np.random.random(100)*5 + 20
In [7]:
# Have a look at the basic form of the data
plt.plot(x, y)
plt.xlabel('X Values')
plt.ylabel('Y Values')
plt.title('My Temperature Data')
Out[7]:
In [8]:
# Let's add some interactive widgets
from ipywidgets import interact
def plot_pseudotemperature(f, A, An, offset):
x = np.linspace(0, 2*np.pi, 100)
y = A * np.sin(f * x) + np.random.random(100) * An + offset
fig = plt.figure()
plt.plot(x, y)
plt.xlabel('X Values')
plt.ylabel('Y Values')
plt.title('My Temperature Data')
plt.show()
interact(plot_pseudotemperature,
f = (0, 10),
A = (1, 5),
An = (1, 10),
offset = (10, 40))
Out[8]:
In [9]:
# We can specify the type of slider, range, and defaults as well
from ipywidgets import FloatSlider, IntSlider
def plot_pseudotemperature2(f, A, An, offset, title):
x = np.linspace(0, 2*np.pi, 100)
y = A * np.sin(f * x) + np.random.random(100) * An + offset
fig = plt.figure()
plt.plot(x, y)
plt.xlabel('X Values')
plt.ylabel('Y Values')
plt.title(title)
plt.show()
interact(plot_pseudotemperature2,
f = IntSlider(min=1, max=7, value=3),
A = FloatSlider(min=1, max=10, value=5),
An = IntSlider(min=1, max=10, value=1),
offset = FloatSlider(min=1, max=40, value=20),
title = 'My Improved Temperature Plot')
Out[9]: